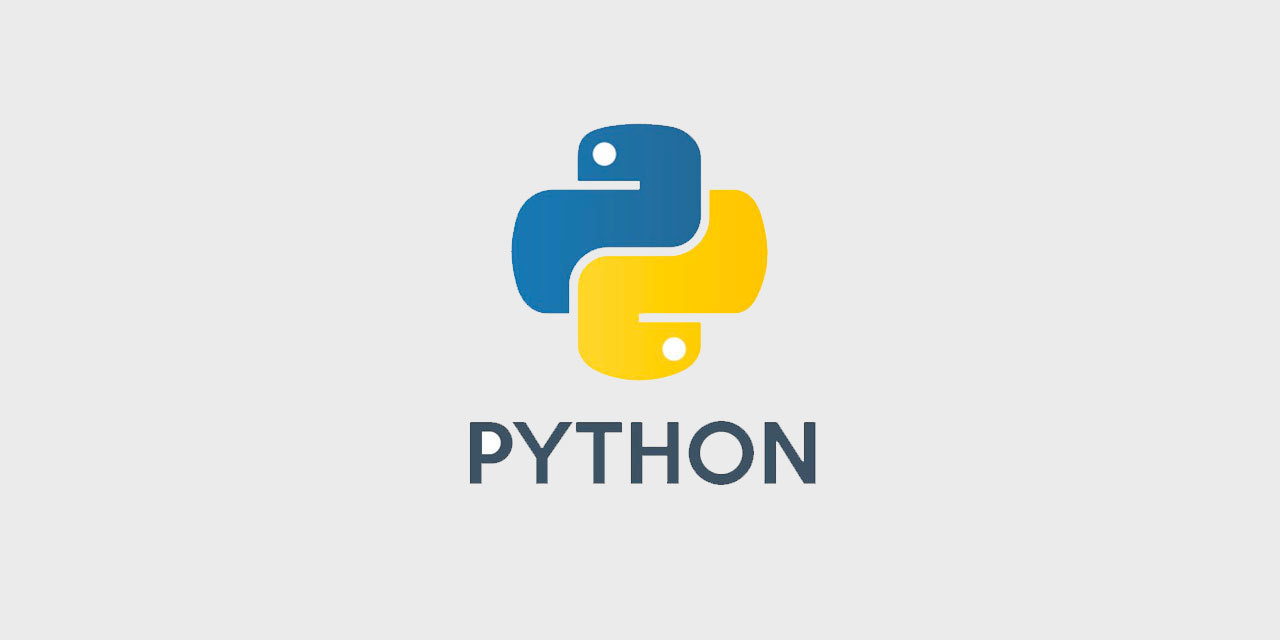
Python学习手册1
Python
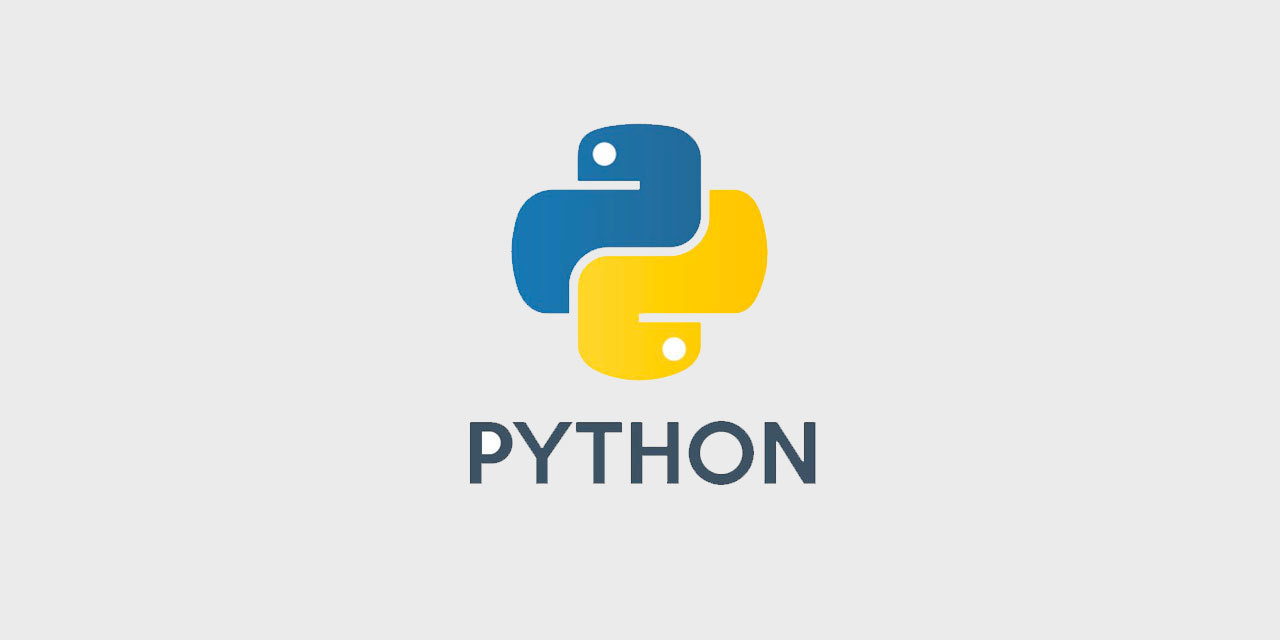
Python基础
Python基础语法
编码
默认情况下,Python 3 源码文件以 UTF-8 编码,所有字符串都是 unicode 字符串。
标识符
- 第一个字符必须是字母表中字母或下划线 _ 。
- 标识符的其他的部分由字母、数字和下划线组成。
- 标识符对大小写敏感。 在 Python 3 中,可以用中文作为变量名,非 ASCII 标识符也是允许的了。
注释
#单行注释
'''
多行注释
'''
python保留字
保留字即关键字,我们不能把它们用作任何标识符名称。Python 的标准库提供了一个 keyword 模块,可以输出当前版本的所有关键字:
>>> import keyword
>>> keyword.kwlist
['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
行与缩进
- python最具特色的就是使用缩进来表示代码块,不需要使用大括号 {} 。
- Python 通常是一行写完一条语句,但如果语句很长,我们可以使用反斜杠 \ 来实现多行语句
- Python 可以在同一行中使用多条语句,语句之间使用分号 ; 分割
Python基本数据类型
Python 中的变量不需要声明。每个变量在使用前都必须赋值,变量赋值以后该变量才会被创建。 在 Python 中,变量就是变量,它没有类型,我们所说的"类型"是变量所指的内存中对象的类型。
标准数据类型
- Number(数字)
- String(字符串)
- bool(布尔类型)
- List(列表)
- Tuple(元组)
- Set(集合)
- Dictionary(字典)
Number(数字)
Python 数字数据类型用于存储数值。 Python 支持三种不同的数值类型:
整型(int) - 通常被称为是整型或整数,是正或负整数,不带小数点。Python3 整型是没有限制大小的,可以当作 Long 类型使用,所以 Python3 没有 Python2 的 Long 类型。布尔(bool)是整型的子类型。
浮点型(float) - 浮点型由整数部分与小数部分组成,浮点型也可以使用科学计数法表示
复数( (complex)) - 复数由实数部分和虚数部分构成,可以用a + bj,或者complex(a,b)表示, 复数的实部a和虚部b都是浮点型。
String(字符串)
字符串是 Python 中最常用的数据类型。使用引号( ' 或 " )来创建字符串。
Python 不支持单字符类型,单字符在 Python 中也是作为一个字符串使用。
Python 访问子字符串,可以使用方括号 [] 来截取字符串,字符串的截取的语法格式如下:
变量[头下标:尾下标]
bool(布尔类型)
布尔类型即 True 或 False。 在 Python 中,True 和 False 都是关键字,表示布尔值。 布尔类型可以用来控制程序的流程,比如判断某个条件是否成立,或者在某个条件满足时执行某段代码。 布尔类型特点:
- 布尔类型只有两个值:True 和 False。
- bool 是 int 的子类,因此布尔值可以被看作整数来使用,其中 True 等价于 1。
- 布尔类型可以和其他数据类型进行比较,比如数字、字符串等。在比较时,Python 会将 True 视为 1,False 视为 0。
- 布尔类型可以和逻辑运算符一起使用,包括 and、or 和 not。这些运算符可以用来组合多个布尔表达式,生成一个新的布尔值。
- 布尔类型也可以被转换成其他数据类型,比如整数、浮点数和字符串。在转换时,True 会被转换成 1,False 会被转换成 0。
- 可以使用 bool() 函数将其他类型的值转换为布尔值。以下值在转换为布尔值时为 False:None、False、零 (0、0.0、0j)、空序列(如 ''、()、[])和空映射(如 {})。其他所有值转换为布尔值时均为 True。
List(列表)
Python 中的 list(列表)是一种有序的可变数据类型,用于存储一组有序的项目。列表中的元素可以是任意类型,包括数字、字符串、列表,甚至是其他复杂对象。以下是关于 Python 列表的一些基本操作和用法:
创建列表
# 创建空列表
empty_list = []
# 创建包含元素的列表
numbers = [1, 2, 3, 4, 5]
strings = ["apple", "banana", "cherry"]
mixed = [1, "apple", 3.14, [2, 4, 6]]
访问列表元素
# 访问列表中的第一个元素(索引从 0 开始)
first_number = numbers[0] # 1
# 访问列表中的最后一个元素
last_number = numbers[-1] # 5
# 访问嵌套列表中的元素
nested_element = mixed[3][1] # 4
修改列表元素
# 修改列表中的元素
numbers[0] = 10 # numbers 现在是 [10, 2, 3, 4, 5]
列表切片
# 获取列表的一部分(切片)
sub_list = numbers[1:4] # [2, 3, 4]
# 切片的步长
stepped_list = numbers[::2] # [10, 3, 5]
添加元素
# 在列表末尾添加元素
numbers.append(6) # [10, 2, 3, 4, 5, 6]
# 在指定位置插入元素
numbers.insert(1, 15) # [10, 15, 2, 3, 4, 5, 6]
删除元素
# 删除指定位置的元素
del numbers[1] # [10, 2, 3, 4, 5, 6]
# 删除并返回最后一个元素
last_element = numbers.pop() # 6, numbers 现在是 [10, 2, 3, 4, 5]
# 删除指定值的元素
numbers.remove(2) # [10, 3, 4, 5]
其他常用方法
# 获取列表长度
length = len(numbers) # 4
# 统计某个元素在列表中出现的次数
count = numbers.count(3) # 1
# 反转列表
numbers.reverse() # [5, 4, 3, 10]
# 排序列表
numbers.sort() # [3, 4, 5, 10]
列表的高级操作
# 列表推导式(列表解析)
squares = [x**2 for x in range(1, 6)] # [1, 4, 9, 16, 25]
# 列表的合并
combined = numbers + squares # [3, 4, 5, 10, 1, 4, 9, 16, 25]
# 列表的重复
repeated = numbers * 2 # [3, 4, 5, 10, 3, 4, 5, 10]
这些操作展示了 Python 列表的基本用法和功能。列表是一种非常灵活和强大的数据结构,适用于各种编程任务。
Tuple(元组)
Python 中的 tuple(元组)是一种有序的不可变数据类型,用于存储一组有序的项目。元组与列表非常相似,但元组一旦创建,其内容不能更改。以下是关于 Python 元组的一些基本操作和用法:
创建元组
# 创建空元组
empty_tuple = ()
# 创建包含元素的元组
numbers = (1, 2, 3, 4, 5)
strings = ("apple", "banana", "cherry")
mixed = (1, "apple", 3.14, (2, 4, 6))
# 不带括号的元组
no_parentheses_tuple = 1, 2, 3, 4, 5
# 创建单个元素的元组(注意逗号)
single_element_tuple = (1,)
访问元组元素
# 访问元组中的第一个元素(索引从 0 开始)
first_number = numbers[0] # 1
# 访问元组中的最后一个元素
last_number = numbers[-1] # 5
# 访问嵌套元组中的元素
nested_element = mixed[3][1] # 4
元组切片
# 获取元组的一部分(切片)
sub_tuple = numbers[1:4] # (2, 3, 4)
# 切片的步长
stepped_tuple = numbers[::2] # (1, 3, 5)
解包元组
# 解包元组中的元素
a, b, c, d, e = numbers
# a = 1, b = 2, c = 3, d = 4, e = 5
# 解包部分元素
first, *rest = numbers
# first = 1, rest = [2, 3, 4, 5]
# 使用 "_" 占位符忽略某些元素
_, second, _, fourth, _ = numbers
# second = 2, fourth = 4
合并与重复元组
# 合并元组
combined = numbers + strings # (1, 2, 3, 4, 5, 'apple', 'banana', 'cherry')
# 重复元组
repeated = numbers * 2 # (1, 2, 3, 4, 5, 1, 2, 3, 4, 5)
其他常用操作
# 获取元组长度
length = len(numbers) # 5
# 检查元素是否存在于元组中
exists = 3 in numbers # True
# 获取元素的索引
index = numbers.index(4) # 3
# 统计某个元素在元组中出现的次数
count = numbers.count(2) # 1
元组的使用场景
- 不可变性:因为元组是不可变的,所以可以用作字典的键,或者用在需要保证数据不变的场景中。
- 解包:元组非常适合用于多变量赋值和解包操作。
- 内存优化:元组的内存占用比列表更少,因此在需要大量存储数据且不修改数据时,使用元组可以提高效率。
示例:函数返回多个值
def get_name_and_age():
return "Alice", 30
name, age = get_name_and_age()
# name = "Alice", age = 30
元组在 Python 中提供了一种简洁、高效的方式来处理不可变的有序数据。
Set(集合)
Python 中的 set(集合)是一种无序的、元素唯一的数据类型。集合主要用于存储不重复的元素,并提供高效的成员检测和基本的集合操作,如并集、交集和差集。以下是关于 Python 集合的一些基本操作和用法:
创建集合
# 创建空集合
empty_set = set()
# 创建包含元素的集合
numbers = {1, 2, 3, 4, 5}
mixed = {1, "apple", 3.14, (2, 4, 6)}
# 使用 set() 函数将其他可迭代对象转换为集合
list_to_set = set([1, 2, 3, 2, 1]) # {1, 2, 3}
string_to_set = set("hello") # {'h', 'e', 'l', 'o'}
添加与删除元素
# 添加元素
numbers.add(6) # {1, 2, 3, 4, 5, 6}
# 删除元素(如果元素不存在会引发 KeyError)
numbers.remove(3) # {1, 2, 4, 5, 6}
# 使用 discard 删除元素(如果元素不存在不会引发错误)
numbers.discard(10) # {1, 2, 4, 5, 6}
# 随机删除并返回一个元素
removed_element = numbers.pop() # 可能是任意一个元素
集合操作
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
# 并集
union_set = set1 | set2 # {1, 2, 3, 4, 5, 6}
# 或者使用 union 方法
union_set = set1.union(set2)
# 交集
intersection_set = set1 & set2 # {3, 4}
# 或者使用 intersection 方法
intersection_set = set1.intersection(set2)
# 差集
difference_set = set1 - set2 # {1, 2}
# 或者使用 difference 方法
difference_set = set1.difference(set2)
# 对称差集(即非公共部分的并集)
symmetric_difference_set = set1 ^ set2 # {1, 2, 5, 6}
# 或者使用 symmetric_difference 方法
symmetric_difference_set = set1.symmetric_difference(set2)
集合的其他常用方法
# 检查元素是否在集合中
exists = 2 in numbers # True
# 获取集合长度
length = len(numbers) # 5
# 清空集合
numbers.clear() # set()
# 复制集合
copied_set = numbers.copy() # {1, 2, 4, 5, 6}
集合推导式
# 创建一个集合,包含 1 到 5 的平方数
squares = {x**2 for x in range(1, 6)} # {1, 4, 9, 16, 25}
示例:集合的应用场景
去重
# 从列表中去除重复元素
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_elements = list(set(original_list)) # [1, 2, 3, 4, 5]
检测集合之间的关系
set1 = {1, 2, 3}
set2 = {1, 2, 3, 4, 5}
# 子集
is_subset = set1 <= set2 # True
# 或者使用 issubset 方法
is_subset = set1.issubset(set2)
# 超集
is_superset = set2 >= set1 # True
# 或者使用 issuperset 方法
is_superset = set2.issuperset(set1)
集合在需要处理唯一元素集合、高效成员检测和基本集合操作的场景中非常有用。集合操作是许多算法和数据处理任务的重要组成部分。
Dictionary(字典)
Python 中的 dictionary(字典)是一种无序的、可变的、元素成对存储的集合。字典中的每个元素由键和值组成,键必须是唯一的。字典主要用于通过键快速查找对应的值。以下是关于 Python 字典的一些基本操作和用法:
创建字典
# 创建空字典
empty_dict = {}
# 创建包含元素的字典
person = {"name": "Alice", "age": 30, "city": "New York"}
# 使用 dict() 函数创建字典
person2 = dict(name="Bob", age=25, city="Los Angeles")
访问字典元素
# 通过键访问值
name = person["name"] # "Alice"
# 使用 get() 方法访问值(如果键不存在,则返回 None 或指定的默认值)
age = person.get("age") # 30
country = person.get("country", "Unknown") # "Unknown"
修改字典元素
# 修改现有键的值
person["age"] = 31 # {"name": "Alice", "age": 31, "city": "New York"}
# 添加新键值对
person["country"] = "USA" # {"name": "Alice", "age": 31, "city": "New York", "country": "USA"}
删除字典元素
# 删除指定键值对
del person["city"] # {"name": "Alice", "age": 31, "country": "USA"}
# 使用 pop() 方法删除并返回指定键的值
age = person.pop("age") # 31, person 现在是 {"name": "Alice", "country": "USA"}
# 使用 popitem() 方法随机删除并返回一个键值对
item = person.popitem() # 例如 ("country", "USA"), person 现在是 {"name": "Alice"}
# 清空字典
person.clear() # {}
字典的其他常用操作
# 获取字典的所有键
keys = person.keys() # dict_keys(["name"])
# 获取字典的所有值
values = person.values() # dict_values(["Alice"])
# 获取字典的所有键值对
items = person.items() # dict_items([("name", "Alice")])
# 检查键是否在字典中
exists = "name" in person # True
not_exists = "age" in person # False
# 字典的长度
length = len(person) # 1
字典推导式
# 创建一个字典,键是 1 到 5,值是它们的平方
squares = {x: x**2 for x in range(1, 6)} # {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
字典的合并与更新
dict1 = {"a": 1, "b": 2}
dict2 = {"b": 3, "c": 4}
# 使用 update() 方法合并字典
dict1.update(dict2) # dict1 现在是 {"a": 1, "b": 3, "c": 4}
# 使用 ** 运算符合并字典(适用于 Python 3.5 及以上)
merged_dict = {**dict1, **dict2} # {"a": 1, "b": 3, "c": 4}
示例:字典的实际应用
统计单词出现的次数
text = "apple banana apple cherry banana apple"
word_counts = {}
for word in text.split():
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
# word_counts 现在是 {"apple": 3, "banana": 2, "cherry": 1}
记录学生成绩
grades = {
"Alice": {"math": 90, "science": 85},
"Bob": {"math": 78, "science": 82},
}
# 访问 Alice 的数学成绩
alice_math_grade = grades["Alice"]["math"] # 90
# 添加一个新学生的成绩
grades["Charlie"] = {"math": 95, "science": 88}
字典在需要快速查找、插入和删除操作的场景中非常有用。字典是 Python 中处理键值对数据的首选数据结构,广泛应用于各种编程任务。
Python变量和常量
在Python中,变量和常量是存储数据的两种方式。
变量
变量是可以在程序的执行过程中改变其值的存储单元。在Python中,变量不需要声明类型,类型会根据赋值自动确定。
变量的定义和使用:
# 定义变量并赋值
x = 10
y = 20
# 使用变量
print(x + y) # 输出30
# 修改变量的值
x = 15
print(x + y) # 输出35
常量
常量是其值在程序的执行过程中不能改变的存储单元。Python没有内置的常量类型,通常使用全大写字母的变量名来表示常量,约定俗成地提醒程序员该值不应被修改。
常量的定义和使用:
# 定义常量
PI = 3.14159
GRAVITY = 9.81
# 使用常量
print("圆的面积:", PI * 5 * 5) # 输出圆的面积:78.53975
print("物体的重量:", 70 * GRAVITY) # 输出物体的重量:686.7
# 尽管可以重新赋值,但不建议这么做
PI = 3.14 # 重新赋值,这不是一个好习惯
print("圆的面积:", PI * 5 * 5) # 输出圆的面积:78.5
变量与常量的区别
值的可变性:
- 变量:值可以在程序执行过程中更改。
- 常量:值在程序执行过程中不应更改。
命名约定:
- 变量:通常使用小写字母和下划线的组合,如
my_variable
。 - 常量:通常使用全大写字母和下划线的组合,如
MY_CONSTANT
。
- 变量:通常使用小写字母和下划线的组合,如
通过正确使用变量和常量,可以提高代码的可读性和维护性。
Python数据类型转换
在Python中,不同的数据类型之间可以进行转换。常见的数据类型转换包括整数、浮点数、字符串、列表、元组、集合和字典。以下是一些常用的数据类型转换方法:
1. 转换为整数
使用 int()
函数将其他数据类型转换为整数。
# 浮点数转换为整数
float_number = 3.14
int_number = int(float_number)
print(int_number) # 输出3
# 字符串转换为整数
string_number = "42"
int_number = int(string_number)
print(int_number) # 输出42
# 布尔值转换为整数
boolean_value = True
int_number = int(boolean_value)
print(int_number) # 输出1
2. 转换为浮点数
使用 float()
函数将其他数据类型转换为浮点数。
# 整数转换为浮点数
int_number = 42
float_number = float(int_number)
print(float_number) # 输出42.0
# 字符串转换为浮点数
string_number = "3.14"
float_number = float(string_number)
print(float_number) # 输出3.14
3. 转换为字符串
使用 str()
函数将其他数据类型转换为字符串。
# 整数转换为字符串
int_number = 42
string_number = str(int_number)
print(string_number) # 输出"42"
# 浮点数转换为字符串
float_number = 3.14
string_number = str(float_number)
print(string_number) # 输出"3.14"
4. 转换为列表
使用 list()
函数将其他可迭代对象(如字符串、元组、集合)转换为列表。
# 字符串转换为列表
string_value = "hello"
list_value = list(string_value)
print(list_value) # 输出['h', 'e', 'l', 'l', 'o']
# 元组转换为列表
tuple_value = (1, 2, 3)
list_value = list(tuple_value)
print(list_value) # 输出[1, 2, 3]
5. 转换为元组
使用 tuple()
函数将其他可迭代对象(如字符串、列表、集合)转换为元组。
# 列表转换为元组
list_value = [1, 2, 3]
tuple_value = tuple(list_value)
print(tuple_value) # 输出(1, 2, 3)
# 字符串转换为元组
string_value = "hello"
tuple_value = tuple(string_value)
print(tuple_value) # 输出('h', 'e', 'l', 'l', 'o')
6. 转换为集合
使用 set()
函数将其他可迭代对象(如字符串、列表、元组)转换为集合。
# 列表转换为集合
list_value = [1, 2, 3, 1, 2]
set_value = set(list_value)
print(set_value) # 输出{1, 2, 3}
# 字符串转换为集合
string_value = "hello"
set_value = set(string_value)
print(set_value) # 输出{'h', 'e', 'l', 'o'}
7. 转换为字典
使用 dict()
函数将其他数据类型转换为字典。通常用于包含键值对的可迭代对象。
# 列表的键值对转换为字典
list_value = [("name", "Alice"), ("age", 25)]
dict_value = dict(list_value)
print(dict_value) # 输出{'name': 'Alice', 'age': 25}
通过这些转换函数,Python提供了灵活的数据类型转换方式,便于在程序中进行各种数据处理和操作。
Python运算符
在Python中,运算符用于执行各种操作,如算术运算、比较运算、逻辑运算等。以下是Python中的常见运算符及其用法:
1. 算术运算符
这些运算符用于执行基本的算术运算。
# 加法
result = 3 + 2 # 结果为5
# 减法
result = 3 - 2 # 结果为1
# 乘法
result = 3 * 2 # 结果为6
# 除法
result = 3 / 2 # 结果为1.5
# 取整除
result = 3 // 2 # 结果为1
# 取余
result = 3 % 2 # 结果为1
# 指数
result = 3 ** 2 # 结果为9
2. 比较运算符
这些运算符用于比较两个值,并返回布尔值(True或False)。
# 等于
result = (3 == 2) # 结果为False
# 不等于
result = (3 != 2) # 结果为True
# 大于
result = (3 > 2) # 结果为True
# 小于
result = (3 < 2) # 结果为False
# 大于等于
result = (3 >= 2) # 结果为True
# 小于等于
result = (3 <= 2) # 结果为False
3. 逻辑运算符
这些运算符用于执行逻辑运算。
# 与
result = (True and False) # 结果为False
# 或
result = (True or False) # 结果为True
# 非
result = not True # 结果为False
4. 位运算符
这些运算符用于按位操作整数。
# 按位与
result = 3 & 2 # 结果为2
# 按位或
result = 3 | 2 # 结果为3
# 按位异或
result = 3 ^ 2 # 结果为1
# 按位取反
result = ~3 # 结果为-4
# 左移
result = 3 << 1 # 结果为6
# 右移
result = 3 >> 1 # 结果为1
5. 赋值运算符
这些运算符用于将值赋给变量。
# 简单赋值
x = 3
# 加法赋值
x += 2 # 相当于x = x + 2,结果为5
# 减法赋值
x -= 2 # 相当于x = x - 2,结果为1
# 乘法赋值
x *= 2 # 相当于x = x * 2,结果为6
# 除法赋值
x /= 2 # 相当于x = x / 2,结果为1.5
# 取整除赋值
x //= 2 # 相当于x = x // 2,结果为1
# 取余赋值
x %= 2 # 相当于x = x % 2,结果为1
# 指数赋值
x **= 2 # 相当于x = x ** 2,结果为9
# 按位与赋值
x &= 2 # 相当于x = x & 2
# 按位或赋值
x |= 2 # 相当于x = x | 2
# 按位异或赋值
x ^= 2 # 相当于x = x ^ 2
# 左移赋值
x <<= 2 # 相当于x = x << 2
# 右移赋值
x >>= 2 # 相当于x = x >> 2
6. 成员运算符
这些运算符用于测试序列中的成员资格。
# in 运算符
result = 'a' in 'apple' # 结果为True
# not in 运算符
result = 'b' not in 'apple' # 结果为True
7. 身份运算符
这些运算符用于比较对象的内存位置。
# is 运算符
x = [1, 2, 3]
y = x
result = x is y # 结果为True
# is not 运算符
y = [1, 2, 3]
result = x is not y # 结果为True
通过理解和使用这些运算符,您可以在Python中执行各种类型的操作,从而实现丰富的功能。
Python循环与流程控制
在Python中,循环与流程控制是编写程序逻辑的基础。以下是一些常见的控制结构和循环结构:
if 条件分支
if
语句用于根据条件执行不同的代码块。
x = 10
if x > 0:
print("x 是正数")
elif x == 0:
print("x 是零")
else:
print("x 是负数")
match 判断
从Python 3.10开始,match
语句被引入,用于模式匹配,这类似于其他语言中的switch语句。
def http_error(status):
match status:
case 400:
return "Bad request"
case 404:
return "Not found"
case 418:
return "I'm a teapot"
case _:
return "Something's wrong with the internet"
print(http_error(404)) # 输出 "Not found"
while 循环
while
循环在条件为真时反复执行代码块。
count = 0
while count < 5:
print(count)
count += 1
for 循环
for
循环用于遍历序列(如列表、元组、字符串)中的元素。
# 遍历列表
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
# 遍历字符串
for char in "hello":
print(char)
中断语句
中断语句用于控制循环的执行,包括 break
、continue
和 else
。
break 语句
break
语句用于提前退出循环。
for num in range(10):
if num == 5:
break
print(num) # 输出0到4
continue 语句
continue
语句用于跳过当前迭代并继续下一次迭代。
for num in range(10):
if num % 2 == 0:
continue
print(num) # 输出1, 3, 5, 7, 9
else 子句
else
子句在循环正常结束时执行,而不是在 break
中断时执行。
for num in range(5):
print(num)
else:
print("循环正常结束") # 输出0到4,然后输出 "循环正常结束"
# 如果在循环中使用break,else子句不会执行
for num in range(5):
if num == 3:
break
print(num)
else:
print("循环正常结束") # 输出0, 1, 2
Python 函数编程
函数是Python编程中的核心组成部分,它们帮助你组织代码、实现复用和提高可读性。以下是Python函数编程的一些关键概念和技术:
函数声明和调用
函数声明用于定义一个函数,包括函数名、参数和函数体。函数调用用于执行函数中的代码。
# 函数声明
def greet(name):
print(f"Hello, {name}!")
# 函数调用
greet("Alice") # 输出: Hello, Alice!
函数的参数
函数可以接受不同类型的参数,包括位置参数、默认参数、关键字参数和可变参数。
# 位置参数
def add(a, b):
return a + b
# 默认参数
def greet(name="Guest"):
print(f"Hello, {name}!")
# 关键字参数
def describe_person(name, age):
print(f"{name} is {age} years old.")
# 可变参数
def sum_numbers(*numbers):
return sum(numbers)
# 调用示例
print(add(2, 3)) # 输出: 5
greet() # 输出: Hello, Guest!
greet("Bob") # 输出: Hello, Bob!
describe_person(age=30, name="Alice") # 输出: Alice is 30 years old.
print(sum_numbers(1, 2, 3, 4)) # 输出: 10
匿名函数
匿名函数(lambda函数)是没有名称的函数,用于简短的函数定义。
# lambda函数
add = lambda x, y: x + y
print(add(2, 3)) # 输出: 5
# 在函数中使用lambda
numbers = [1, 2, 3, 4]
squared = list(map(lambda x: x**2, numbers))
print(squared) # 输出: [1, 4, 9, 16]
递归
递归是指函数在其定义中调用自身。递归函数必须有一个基本条件以防止无限递归。
# 计算阶乘的递归函数
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # 输出: 120
高阶函数
高阶函数是接受函数作为参数或返回函数的函数。
# 使用高阶函数map
numbers = [1, 2, 3, 4]
squared = list(map(lambda x: x**2, numbers))
print(squared) # 输出: [1, 4, 9, 16]
# 高阶函数返回函数
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
add_five = outer_function(5)
print(add_five(3)) # 输出: 8
返回函数和闭包
闭包是指一个函数返回另一个函数,且内部函数可以访问外部函数的局部变量。
# 闭包示例
def make_multiplier(factor):
def multiplier(number):
return number * factor
return multiplier
# 创建闭包
times_three = make_multiplier(3)
print(times_three(9)) # 输出: 27
函数装饰器
装饰器是一种用于修改或增强函数的功能的设计模式。它是一个函数,接受另一个函数作为参数,并返回一个新的函数。
def decorator_function(original_function):
def wrapper_function():
print("Wrapper executed this before {}".format(original_function.__name__))
return original_function()
return wrapper_function
@decorator_function
def display():
return "Display function executed"
print(display()) # 输出: Wrapper executed this before display
# 输出: Display function executed
偏函数
偏函数使用 functools.partial
创建,允许你固定函数的部分参数。
from functools import partial
# 偏函数
def power(base, exponent):
return base ** exponent
# 创建偏函数
square = partial(power, exponent=2)
print(square(4)) # 输出: 16
cube = partial(power, exponent=3)
print(cube(2)) # 输出: 8
高级特性
切片
切片(Slicing)允许你从序列(如列表、元组、字符串)中提取子集。切片操作使用 start:stop:step
语法。
# 列表切片
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(numbers[2:5]) # 输出: [2, 3, 4]
print(numbers[:5]) # 输出: [0, 1, 2, 3, 4]
print(numbers[5:]) # 输出: [5, 6, 7, 8, 9]
print(numbers[::2]) # 输出: [0, 2, 4, 6, 8]
print(numbers[::-1]) # 输出: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
# 字符串切片
text = "Hello, World!"
print(text[7:12]) # 输出: World
推导式
推导式(Comprehensions)是一种简洁的创建列表、集合、字典等的方式。
列表推导式
# 创建一个包含1到10平方数的列表
squares = [x**2 for x in range(1, 11)]
print(squares) # 输出: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
集合推导式
# 创建一个包含1到10平方数的集合
squares_set = {x**2 for x in range(1, 11)}
print(squares_set) # 输出: {64, 1, 4, 36, 9, 100, 16, 81, 49, 25}
字典推导式
# 创建一个键为数字,值为该数字平方的字典
squares_dict = {x: x**2 for x in range(1, 11)}
print(squares_dict) # 输出: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81, 10: 100}
迭代器
迭代器(Iterator)是一个实现了 __iter__()
和 __next__()
方法的对象,允许你遍历容器中的所有元素。
# 创建一个简单的迭代器
class MyIterator:
def __init__(self, limit):
self.limit = limit
self.counter = 0
def __iter__(self):
return self
def __next__(self):
if self.counter < self.limit:
self.counter += 1
return self.counter
else:
raise StopIteration
# 使用迭代器
iterator = MyIterator(5)
for num in iterator:
print(num) # 输出: 1 2 3 4 5
生成器
生成器(Generator)是一种特殊的迭代器,用 yield
语句返回值,保持函数的状态以供下次迭代。
# 创建一个生成器函数
def simple_generator():
yield 1
yield 2
yield 3
# 使用生成器
for value in simple_generator():
print(value) # 输出: 1 2 3
装饰器
装饰器(Decorator)是一种用于修改或增强函数功能的设计模式。它是一个接受函数并返回一个新函数的函数。
# 定义一个简单的装饰器
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
result = func()
print("Something is happening after the function is called.")
return result
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
# 输出:
# Something is happening before the function is called.
# Hello!
# Something is happening after the function is called.
Python模块
Python模块使你能够将代码分割成多个文件,以便更好地组织和复用代码。
使用模块
模块是一个包含Python定义和语句的文件。模块可以包含函数、类和变量,还可以包含可执行的代码。
导入模块
使用 import
语句导入模块。
# 导入整个模块
import math
print(math.sqrt(16)) # 输出: 4.0
# 导入模块中的特定函数
from math import sqrt
print(sqrt(16)) # 输出: 4.0
# 导入模块并给它起一个别名
import math as m
print(m.sqrt(16)) # 输出: 4.0
安装模块
使用 pip
工具从Python包索引(PyPI)安装第三方模块。
# 安装一个模块
pip install requests
# 卸载一个模块
pip uninstall requests
# 查看已安装的模块列表
pip list
内置模块
Python自带了一些非常有用的内置模块,以下是一些常见的内置模块:
os
模块
提供了一种便携的方式使用操作系统功能。
import os
# 获取当前工作目录
print(os.getcwd())
# 列出当前目录下的文件和目录
print(os.listdir())
# 创建新目录
os.mkdir('new_directory')
# 删除目录
os.rmdir('new_directory')
sys
模块
提供了对解释器使用或维护的一些变量和函数的访问。
import sys
# 获取命令行参数
print(sys.argv)
# 退出程序
sys.exit()
datetime
模块
用于处理日期和时间。
import datetime
# 获取当前日期和时间
now = datetime.datetime.now()
print(now)
# 创建一个特定日期
date = datetime.date(2023, 1, 1)
print(date)
# 计算两个日期之间的差异
delta = datetime.date(2023, 1, 1) - datetime.date(2022, 1, 1)
print(delta.days)
random
模块
用于生成随机数。
import random
# 生成一个0到1之间的随机浮点数
print(random.random())
# 生成一个指定范围内的随机整数
print(random.randint(1, 10))
# 从列表中随机选择一个元素
choices = ['apple', 'banana', 'cherry']
print(random.choice(choices))
常用模块
除了内置模块,还有一些常用的第三方模块:
requests
模块
用于发送HTTP请求。
import requests
response = requests.get('https://api.github.com')
print(response.status_code)
print(response.json())
numpy
模块
用于科学计算。
import numpy as np
# 创建一个数组
array = np.array([1, 2, 3, 4])
print(array)
# 创建一个二维数组
matrix = np.array([[1, 2], [3, 4]])
print(matrix)
pandas
模块
用于数据分析和处理。
import pandas as pd
# 创建一个数据框
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
# 从CSV文件读取数据
df = pd.read_csv('data.csv')
print(df)
matplotlib
模块
用于绘制图形和可视化数据。
import matplotlib.pyplot as plt
# 创建简单的折线图
x = [1, 2, 3, 4]
y = [1, 4, 9, 16]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
plt.show()
Python面向对象编程
面向对象编程(OOP)是一种编程范式,它将程序结构化为对象的集合,每个对象包含数据和操作这些数据的方法。在Python中,面向对象编程的核心概念包括类、对象、继承、封装和多态。
核心概念
类(Class): 类是对象的蓝图或模板。它定义了一组属性和方法,这些属性和方法将被用于创建对象。
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): print(f"{self.name} is barking!")
对象(Object): 对象是类的实例。创建对象的过程称为实例化。
my_dog = Dog("Buddy", 3) my_dog.bark() # 输出: Buddy is barking!
继承(Inheritance): 继承是一种创建新类的方式,新类继承自现有类,可以重用、扩展和修改父类的行为。
class Animal: def __init__(self, name): self.name = name def speak(self): pass class Dog(Animal): def speak(self): return f"{self.name} says Woof!" my_dog = Dog("Buddy") print(my_dog.speak()) # 输出: Buddy says Woof!
封装(Encapsulation): 封装是一种将数据和方法捆绑在一起,并隐藏对象的内部实现细节的方式。通过使用私有属性(以双下划线开头),可以实现封装。
class Person: def __init__(self, name, age): self.__name = name self.__age = age def get_name(self): return self.__name def get_age(self): return self.__age person = Person("Alice", 30) print(person.get_name()) # 输出: Alice
多态(Polymorphism): 多态是指不同类的对象可以通过相同的接口调用方法,尽管这些方法的具体实现可能不同。
class Cat(Animal): def speak(self): return f"{self.name} says Meow!" animals = [Dog("Buddy"), Cat("Kitty")] for animal in animals: print(animal.speak()) # 输出: Buddy says Woof! # Kitty says Meow!
示例
以下是一个简单的Python面向对象编程示例,展示了类、对象、继承、封装和多态的使用:
class Animal:
def __init__(self, name):
self.__name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
def get_name(self):
return self.__name
class Dog(Animal):
def speak(self):
return f"{self.get_name()} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.get_name()} says Meow!"
animals = [Dog("Buddy"), Cat("Kitty")]
for animal in animals:
print(animal.speak())
错误、调试和测试
在编程过程中,错误、调试和测试是确保代码质量和正确性的关键步骤。
常见的错误类型
语法错误(Syntax Error): 代码的语法不符合Python的语法规则,导致代码无法运行。
if True print("Hello, world!") # 缺少冒号(:)
运行时错误(Runtime Error): 代码在运行时发生错误,如除零错误、索引越界等。
result = 10 / 0 # 除零错误
逻辑错误(Logic Error): 代码没有按预期工作,但不会抛出错误。这类错误通常需要通过调试和测试来发现。
def add(a, b): return a - b # 逻辑错误,应该是 a + b
调试技巧
使用
print
语句: 在关键位置插入print
语句,查看变量的值和程序的执行流程。def add(a, b): print(f"a: {a}, b: {b}") return a + b result = add(2, 3) print(result)
使用断点和调试器: 使用集成开发环境(IDE)中的调试工具,如PyCharm、VS Code等,设置断点并逐步执行代码。
使用
pdb
模块: Python内置的调试器,可以在代码中插入断点,交互式调试。import pdb def add(a, b): pdb.set_trace() # 插入断点 return a + b result = add(2, 3) print(result)
测试方法
单元测试(Unit Testing): 测试代码中的独立单元,如函数或类方法,确保它们按预期工作。Python中的
unittest
模块是一个常用的单元测试框架。import unittest def add(a, b): return a + b class TestMathFunctions(unittest.TestCase): def test_add(self): self.assertEqual(add(2, 3), 5) self.assertEqual(add(-1, 1), 0) self.assertEqual(add(0, 0), 0) if __name__ == "__main__": unittest.main()
测试驱动开发(TDD): 先编写测试用例,再编写实现代码。通过不断运行测试来驱动代码的开发。
集成测试(Integration Testing): 测试多个组件或模块之间的交互,确保它们能够协同工作。
功能测试(Functional Testing): 测试系统的功能是否符合需求,通过模拟用户操作来验证系统行为。
示例:调试和测试
假设你有一个简单的类,用于表示一个人的信息,并实现一个方法来获取其全名:
class Person:
def __init__(self, first_name, last_name):
self.first_name = first_name
self.last_name = last_name
def get_full_name(self):
return f"{self.first_name} {self.last_name}"
import unittest
class TestPerson(unittest.TestCase):
def test_get_full_name(self):
person = Person("John", "Doe")
self.assertEqual(person.get_full_name(), "John Doe")
if __name__ == "__main__":
unittest.main()
在调试时,你可以在get_full_name
方法中加入print
语句查看first_name
和last_name
的值:
class Person:
def __init__(self, first_name, last_name):
self.first_name = first_name
self.last_name = last_name
def get_full_name(self):
print(f"First Name: {self.first_name}, Last Name: {self.last_name}")
return f"{self.first_name} {self.last_name}"
通过单元测试确保get_full_name
方法的正确性,并使用调试技巧找到并修复潜在的错误。这种方法可以帮助你在研究中编写更可靠、更高效的代码。
文件与IO
在Python中,文件和输入输出(IO)操作是处理数据的基本功能。了解如何读取和写入文件、处理各种文件格式,以及如何有效地进行文件操作是非常重要的。
文件操作基础
打开文件
在Python中,使用open()
函数打开文件。该函数返回一个文件对象,可以用来读写文件。常见的模式包括:
'r'
:只读(默认)'w'
:写入(会截断文件)'a'
:追加'b'
:二进制模式't'
:文本模式(默认)
# 打开文件进行读取
file = open('example.txt', 'r')
# 打开文件进行写入
file = open('example.txt', 'w')
读取文件
可以使用多种方法读取文件内容:
read(size)
:读取整个文件或指定大小的字节数readline()
:读取一行readlines()
:读取所有行并返回列表
with open('example.txt', 'r') as file:
content = file.read()
print(content)
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
写入文件
可以使用write()
和writelines()
方法将数据写入文件。
with open('example.txt', 'w') as file:
file.write("Hello, World!\n")
with open('example.txt', 'a') as file:
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
file.writelines(lines)
关闭文件
虽然使用with
语句可以自动关闭文件,但也可以手动关闭文件。
file = open('example.txt', 'r')
content = file.read()
file.close()
文件路径
使用os
模块处理文件路径,使代码更具可移植性。
import os
# 获取当前工作目录
current_dir = os.getcwd()
print(current_dir)
# 连接路径
file_path = os.path.join(current_dir, 'example.txt')
print(file_path)
文件和目录操作
使用os
模块可以执行各种文件和目录操作,如重命名、删除和创建目录。
import os
# 重命名文件
os.rename('old_name.txt', 'new_name.txt')
# 删除文件
os.remove('example.txt')
# 创建目录
os.mkdir('new_directory')
# 删除目录
os.rmdir('new_directory')
处理CSV文件
使用csv
模块读写CSV文件。
import csv
# 写入CSV文件
with open('example.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
# 读取CSV文件
with open('example.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
处理JSON文件
使用json
模块读写JSON文件。
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
# 写入JSON文件
with open('example.json', 'w') as jsonfile:
json.dump(data, jsonfile)
# 读取JSON文件
with open('example.json', 'r') as jsonfile:
data = json.load(jsonfile)
print(data)
示例
以下是一个综合示例,展示了如何读取文本文件内容,处理数据并写入到新的CSV文件中:
import csv
# 读取文本文件
with open('data.txt', 'r') as file:
lines = file.readlines()
# 处理数据
data = [line.strip().split() for line in lines]
# 写入CSV文件
with open('output.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Column1', 'Column2', 'Column3'])
writer.writerows(data)
在Python中,输入和输出(I/O)操作是与用户进行交互、处理文件以及与其他程序通信的基本功能。以下是Python中常见的I/O操作,包括标准输入输出和文件I/O。
标准输入输出
标准输出
标准输出通常指将信息显示在屏幕上,使用print()
函数实现。
# 输出字符串
print("Hello, World!")
# 输出多个值
print("The answer is", 42)
# 使用格式化字符串
name = "Alice"
age = 30
print(f"My name is {name} and I am {age} years old.")
标准输入
标准输入通常指从用户获取输入,使用input()
函数实现。input()
函数总是返回一个字符串。
# 获取用户输入
name = input("Enter your name: ")
print(f"Hello, {name}!")
# 获取数值输入
age = int(input("Enter your age: "))
print(f"You are {age} years old.")